Using lists() in Laravel with custom attribute accessors
This blog post was originally published a little while ago. Please consider that it may no longer be relevant or even accurate.
Sometimes when you're listing out a model for a select box or another form of displaying your data, you'll want to use a custom format for the list and keep the key as the row ID. You might want to get your collection first and then loop through it to create the array in the format you need, but there is a better way.
For example in a project I'm working on we want to list out all the subjects of a university displaying both the subject code and it's name. The way we go about this is to define a custom attribute getter on our Subject
Eloquent model:
Now, when we have a Subject
model with a code of "ARTS3091" and a name of "Advanced Media Issues", we can get "ARTS3091: Advanced Media Issues" simply by calling the $subject->full_name
attribute. Easy!
But how does this work when we want to list out subjects from the database - there isn't a full_name
attribute in the database!
If you tried that, you'll see it will fail for that very reason...
This is because lists()
on the model will look up on the database. What we want is for lists()
to look through a collection of models, that way each model will have the attribute that can be used!
Works a charm! So, when you're trying to list out using a custom accessor (to achieve a certain format or other reason), be sure to call it on a collection rather than directly on the model!
A blog about Laravel & Rails by Dwight Watson;
- developer of Roomies, High School Notes & StudentVIP.
- previously myRent & Flatmates.
- also writing Minitest on Rails.
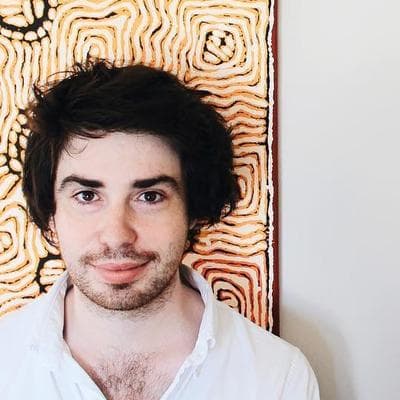